Your Ultimate Guide to Docker: Commands, Components, and Interview Prep
In today’s DevOps-driven world, Docker has revolutionized how we build, ship, and run applications.
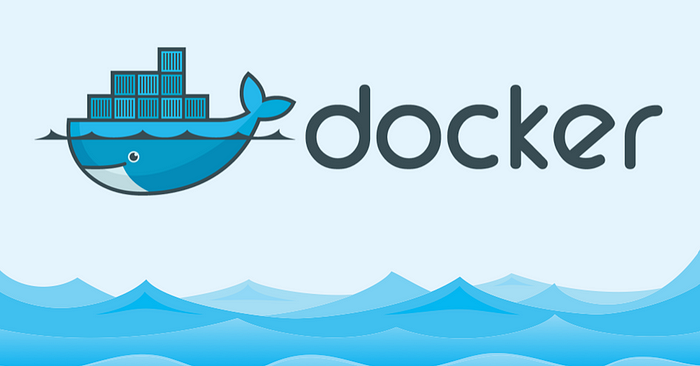
🚢 Why Docker?
Docker is a platform that uses containerization to simplify application development and deployment. Imagine shipping an app along with its environment in a tiny, portable box — that’s Docker for you. No more “It works on my machine” excuses!
🛠️ Essential Docker Commands
Run a Container:
docker run hello-world
This pulls the hello-world
image, creates a container, and runs it. It’s the Docker equivalent of saying, “Hello, containers!”
List Running Containers:
docker ps
See all active containers at a glance. Add -a
to see stopped ones too.
Stop a Container:
docker stop <container-id>
Gracefully shuts down the container.
Remove a Container:
docker rm <container-id>
Clear out those stopped containers to free up space.
2. Image Management
Pull an Image:
docker pull nginx
Downloads the latest NGINX image.
Build an Image:
docker build -t my-app:1.0 .
Creates an image from a Dockerfile
in the current directory.
Push an Image to Docker Hub:
docker tag my-app:1.0 my-dockerhub-username/my-app:1.0
docker push my-dockerhub-username/my-app:1.0
Tags and uploads your image to Docker Hub.
Remove an Image:
docker rmi <image-id>
Cleans up unused images.
3. System Cleanup
Remove Unused Data:
docker system prune
Cleans up unused containers, networks, images, and build cache.
Remove Stopped Containers:
docker container prune
Clears out all stopped containers.
4. Advanced Commands
Execute a Command Inside a Running Container:
docker exec -it <container-id> /bin/bash
Gives you an interactive shell inside the container.
Copy Files Between Host and Container:
docker cp <source-path> <container-id>:<destination-path>
docker cp <container-id>:<source-path> <destination-path>
Facilitates moving files to and from containers.
Commit Changes to a Container:
docker commit <container-id> my-new-image:1.0
Saves the current state of a container as a new image.
Port Binding:
docker run -p 8080:80 nginx
Maps port 80 inside the container to port 8080 on the host.
Detached Mode:
docker run -d nginx
Runs the container in the background.
📄 Dockerfile Components
A Dockerfile
is the blueprint for your container image. Here’s a breakdown:
# 1. Base Image
FROM python:3.9-slim
# 2. Set Working Directory
WORKDIR /app
# 3. Copy Files
COPY requirements.txt .
# 4. Install Dependencies
RUN pip install -r requirements.txt
# 5. Add Application Code
COPY . .
# 6. Expose Port
EXPOSE 5000
# 7. Specify Start Command
CMD ["python", "app.py"]
Key Instructions:
- FROM: Specifies the base image.
- WORKDIR: Sets the working directory inside the container.
- COPY vs ADD:
COPY
is used to copy files from the host to the image.ADD
can also fetch files from remote URLs and extract tar archives.- RUN: Executes commands during image creation, creating a new layer.
- CMD vs ENTRYPOINT: In a Dockerfile,
CMD
andENTRYPOINT
are instructions that specify what command to run when a container is started. CMD
provides default commands and arguments.ENTRYPOINT
is used to define the container’s main process, making it immutable.
📦 Docker Compose File Components
Docker Compose simplifies multi-container setups with a single YAML file:
version: "3.9"
services:
app:
build:
context: .
ports:
- "5000:5000"
volumes:
- .:/app
networks:
- my-network
db:
image: postgres:latest
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
networks:
- my-network
networks:
my-network:
driver: bridge
Key Components:
- services: Define each containerized app.
- build: Specifies the context and
Dockerfile
for image creation. - ports: Maps host ports to container ports.
- volumes: Mounts directories for persistent storage.
- networks: Configures communication between containers.
🧩 Docker Components Demystified
1. Images: Pre-packaged environments for running applications.
2. Containers: Running instances of images — like apps with their own environments.
3. Volumes: Persistent data storage for containers.
4. Networks: Isolated communication channels for containers.
5. Docker Daemon: The background service that powers it all.
6. Docker CLI: The command-line interface for interacting with Docker.
🎯 Most Asked Docker Interview Questions
Q1: What is Docker?
Docker is a open-source containerization platform that packages applications with their dependencies, ensuring consistency across environments.
Q2: What’s the difference between a Docker image and a container?
Docker image: A read-only template that contains the application code, libraries, dependencies, and configurations needed to run an application. It is a blueprint used to create Docker containers.
Think of it as a “snapshot” of the application and its environment at a specific point in time.
Docker Container: A runtime instance of a Docker image. It represents the actual execution of the application in an isolated environment and allows for changes (e.g., writing files, modifying configurations) during its runtime.
Q3: What is the purpose of docker-compose
?
To manage multi-container Docker applications using a YAML file.
Q4: What is a Docker Volume?
Volumes provide persistent storage independent of container lifecycles.
Q5: How do you optimize Docker images?
1. Use a Smaller Base Image
- Start with a minimal base image like
alpine
, which is just 5 MB, instead of larger images likeubuntu
ordebian
. - Example:
FROM alpine:latest
2. Use a Smaller Base Image
Combine RUN
instructions and avoid unnecessary files.
Example:
RUN apt-get update && apt-get install -y \
curl \
unzip && \
apt-get clean && rm -rf /var/lib/apt/lists/*
3. Use a Smaller Base Image
- Use multi-stage builds to separate the build environment from the final runtime environment, keeping only the necessary artifacts in the final image.
Example:
# Stage 1: Build
FROM golang:1.18 AS builder
WORKDIR /app
COPY . .
RUN go build -o myapp .
# Stage 2: Final image
FROM alpine:latest
WORKDIR /app
COPY --from=builder /app/myapp .
CMD ["./myapp"]
Q6: What is the difference between CMD and ENTRYPOINT?
1. CMD (Command)
- If a command is passed during
docker run
, it overrides theCMD
. - It is not executable by default, meaning it allows users to replace it at runtime.
- Syntax: Executes the command directly as an executable.
CMD ["echo", "Hello, World!"]
- Use Case: Use
CMD
when you want to provide a default command that can easily be overridden.
2. ENTRYPOINT
- The command provided at runtime using
docker run
is appended to theENTRYPOINT
arguments. - It is executable by default, making it more rigid compared to
CMD
. - Syntax:
ENTRYPOINT ["echo"]
- Use Case: Use
ENTRYPOINT
when you want the container to run as a dedicated application or script and enforce a specific command.
3. Combining CMD and ENTRYPOINT
- You can use both
CMD
andENTRYPOINT
together: ENTRYPOINT
defines the base command.CMD
provides the default arguments to theENTRYPOINT
, which can be overridden at runtime.- Example:
FROM ubuntu:latest
ENTRYPOINT ["echo"]
CMD ["Hello, World!"]
Default behavior:
$ docker run myimage
-> Hello, World!
- Overriding
CMD
:
$ docker run myimage Docker!
-> Docker!
Q7: What is the difference between ADD and COPY in a Dockerfile?
ADD
supports downloading from URLs and auto-extracting archives.COPY
is more straightforward and should be used unlessADD
features are needed.
Q8: What happens when you run a RUN
command in a Dockerfile?
It executes during the image build process, creating a new intermediate image layer.
Q9: What are the steps to build and push an image to Docker Hub?
- Write a
Dockerfile
. - Build the image:
docker build -t <image-name> .
- Tag the image:
docker tag <image-name> <dockerhub-username>/<image-name>:<tag>
- Push the image:
docker push <dockerhub-username>/<image-name>:<tag>
🚀 Wrapping It Up
Docker is a powerhouse for modern application development, and mastering its commands, files, and concepts is crucial for anyone in DevOps. This guide has equipped you with the essentials — from practical commands to interview prep. Now, go build something amazing and crush those interviews!
Got questions? Drop them in the comments below. Happy Dockering! 🐳